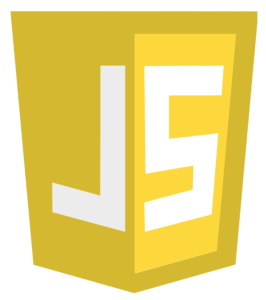
JavaScript Training
This Course Covers Version(s): JavaScript ES6
- Live Class with Instructor
- Digital Course Manual
- Hands-on Labs
- One Year Access to Recorded Course
In addition to HTML and CSS, JavaScript represents one of the main tools web developers use to create rich, responsive web and mobile applications. Its use has expanded with the use of jQuery and AJAX and has evolved JavaScript to become much more than just a scripting language used to manipulate the user interface. In this three-day JavaScript Training course, students will learn to use the most recent, widely supported version of JavaScript. They will learn how to create basic statements in JavaScript, how to declare and use primitive and complex variables and data types (including objects), how to create and use Functions and Expressions and how to match patterns using Regular Expressions. In addition, students will see how to manipulate the Document Object Model (DOM), how to handle keyboard and screen events, how to validate form input with JavaScript and how to debug and handle errors in their JavaScript code.
Upon successful completion of this course, students will be able to:
- Use features of the most recent version of JavaScript
- Use complex and primitive variables and data types
- Work with Objects in JavaScript
- Use Regular Expressions for pattern matching
- Handle and respond to screen and keyboard events
- Validate form input with JavaScript
Students should have familiarity with HTML and CSS. Some programming experience/familiarity is helpful but not a requirement.
This course is designed for Web designers and others who want to learn how to use JavaScript to enhance their Web sites.
- Introduction to JavaScript
- Versions of JavaScript
- Creating your first JavaScript page
- Scripting Terminology
- Expression
- Variable
- Operators
- Statement
- Program or Script
- Language Fundamentals
- Declaring Variables
- Assigning or Setting Variables
- Case Sensitive
- Spacing and Ending Statements
- Comments
- Data Types
- Numeric Variables
- String Variables
- Boolean Variables
- Other Basic Data Types
- Built-in Objects
- Math
- Date
- User input methods
- JavaScript Conditions
- Conditional Constructs
- The if Construct
- Testing Multiple Conditions
- Exercise 2-1 Using the if construct
- The switch Construct
- Exercise 2-2 Using the switch
- The Conditional (Ternary) Operator
- Exercise 2-3 Using the ternary operator
- JavaScript Loops
- JavaScript Loops
- The while Loop
- JavaScript Labels and Branch Statements
- Primitive Data Types
- The String Data Type
- String Properties
- String Methods
- The Number Data Type
- Number Properties
- Number Methods
- Global Functions Used with Numbers
- Compound Data Types
- Arrays
- Storing Data in Arrays
- Array Properties
- Looping Over an Array
- Exercise 5-1 Holding data in an array
- Array Mutator Methods
- Array Accessor Methods
- Multidimensional Arrays
- Objects
- Storing Data in an Object
- Accessing Data in an Object
- Looping Through Data in an Object
- Creating an Object Method
- Creating an Object Constructor
- Arrays of Objects
- Exercise 5-3 Working with objects
- Using JSON Syntax to Store Data
- JSON.parse()
- JSON.stringify()
- Functions
- Declaring and Invoking a Named Function
- Returning Values
- Creating an Anonymous Function Expression
- Scope
- Local and Global Variables
- Function Scope
- Function Arguments
- Setting Default Arguments
- The arguments and this Properties
- Regular Expressions
- Constructing a Regular Expression
- Creating a Pattern
- Using Parentheses to Match Substrings
- Applying Regular Expression Methods
- Regular Expression Method exec()
- Regular Expression Method test()
- Using Regular Expressions in String Methods
- String Method match()
- String Method search()
- String Method replace()
- String Method split()
- Backreferences
- The Browser Object Model
- The Browser Object Model
- The history Object
- The location Object
- The screen Object
- The console Object
- The window Object’s open() and close()Methods
- Using BOM Methods to Execute Code
- setTimeout()/clearTimeout()
- setInterval()/clearInterval()
- The Document Object Model
- The Document Object Model API
- The document Object
- The document Object Access Methods
- Using Selectors to Access Elements
- Working with DOM Nodes
- Creating Nodes with the DOM
- Using the Node Interface Properties
- The Node Traversal Properties
- The Node Methods
- Emulating Methods
- Test Methods
- The Element Interface
- Manipulating CSS with the DOM
- Event Handling
- The event Object
- Event Handling
- Traditional Event Registration
- Registering With addEventListener()
- Removing the Event Listener
- Types of Events
- Mouse Events
- Keyboard Events
- Clipboard Events
- Window Events
- Form Events
- Event Methods
- stopPropagation();
- preventDefault()
- Exercise 10-1 Using events
- Working with forms
- Validating Form Data with JavaScript
- Form Events
- Checking for Empty Fields
- Testing for String Length
- Making Sure a Selection is Made
- Testing whether the user has checked a box
- Data Validation with Regular Expressions
- Debugging and best practices
- JavaScript Coding Styles
- Variable and Statement Coding Styles
- Block Coding Styles
- JavaScript Best Practices
- Optimizing Code Performance
- The “use strict”; Directive
- The JavaScript debugger; Statement
- Custom Error Handling
This course does not align to a specific exam or certification.
- For Private Groups as small as 2 people.
- Live, Instructor-led Online or Onsite Class for your group.
- Customizable to your needs.