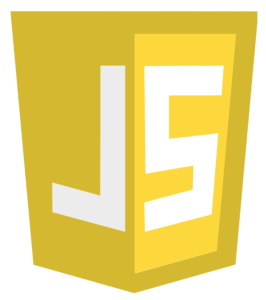
Advanced JavaScript Training
This Course Covers Version(s): JavaScript 6 / ES6
- Live Class with Instructor
- Digital Course Manual
- Hands-on Labs
- Up to 1 Year Access to Recorded Course
In this Advanced JavaScript Programming class, students will learn advanced JavaScript techniques including how to use JavaScript design patterns, how to use JSON and AJAX, how to use Web Storage and Web Workers, how to implement JavaScript in conjunction with HTML5 APIs – including using the Canvas element, what the new features of ECMAScript 6 are and how to use them, what JavaScript Libraries and Frameworks are available and how to utilize them and how to debug JavaScript code and use best practices to optimize your code.
Upon successful completion of this course, students will be able to:
- Use JavaScript design patterns
- Understand and use JSON and AJAX
- Use JavaScript in HTML5 APIs
- Use new features of ECMAScript 6
- Describe JavaScript libraries and Frameworks like Node, Angular and more
- Debug and optimize code using best practices
Completion of, or familiarity with, the topics covered in our JavaScript Training Course.
This course is designed for Web designers and others who have a basic understanding of JavaScript and want to learn about more advanced JavaScript and DHTML functionality.
- JavaScript Design Patterns
- Review of JavaScript Fundamentals
- JavaScript Types
- Variables
- Operators
- Control Structures
- Objects and Arrays
- Functions
- Functional Programming with IIFE
- Object-Oriented JavaScript Programming
- Class
- Constructor
- Object
- Property
- Method
- Namespace
- Inheritance
- Encapsulation
- Polymorphism
- JavaScript Design Patterns
- Constructor Pattern
- Module Pattern
- Singleton Pattern
- Prototype Pattern
- Observer/Publish-Subscribe Pattern
- Factory Pattern
- MV* Patterns
- The MVC Pattern
- The MVVM Pattern
- Templating and Views
- JSON and AJAX
- Introduction to JSON syntax
- JSON Methods
- JSON.stringify()
- JSON.parse()
- Persisting JSON in Web Storage
- AJAX
- Overview of JavaScript in HTML5 APIs
- Web Storage API
- Checking for Web Storage support
- length Property of Web Storage
- setItem() Method of Web Storage
- getItem() Method of Web Storage
- removeItem() and clear() Methods of Web Storage
- key() Method of Web Storage
- Web Workers and Cross-Document Messaging APIs
- Checking for Web Workers support
- Constructing a Web Worker
- Using Cross-Document Messaging for a Web Worker
- Constructing a Web Worker
- Stopping a Web Worker
- Combining the Cross-Document Messaging and Web Worker APIs
- Drag and Drop API
- Creating a draggable element
- Setting dataTransfer information at the ondragstart event
- Specifying an alternative drag image
- Designating a drop target
- Making the target droppable
- Getting transferred data on the drop event
- Canvas API Basics
- Setting the <canvas> tag in the HTML page
- Providing fallback content
- Required closing tag
- The 2D rendering context
- Checking for Canvas API support
- Drawing rectangles and squares
- Alternate method for drawing rectangles and squares
- Drawing circles
- Drawing semi-circles
- Drawing custom shapes
- Best practice: setting a beginning and end to the shape’s path
- Drawing multiple shapes
- Adding text
- Adding shadows
- Adding an image
- Animating the canvas
- ECMAScript 6
- Scoping and Modules
- Lexical Variable Scoping
- Block Scoping
- ES6 Modules
- Iterators
- Arrow Functions and Destructuring Assignment
- Arrow Functions
- Destructuring Assignment
- Literals and Constants
- Template Literals
- Constants
- Parameter Handling
- Default Parameter Values
- Spread Operator
- Rest Parameter
- New Built-in Methods and Internationalization Formatting
- New Array Methods
- New String Methods
- New Number Methods
- Internationalization and Localization Formatting
- Classes
- Defining Classes
- Getter/Setters
- Using a Derived Class to Create a Base Class
- Using super in Classes
- Static Methods
- Map/Set and WeakMap/WeakSet
- Map
- Iterating Maps with for/of
- Set
- WeakMap/WeakSet
- Asynchronous Flow Control
- Promises
- JavaScript Libraries and Frameworks
- Overview of JavaScript Libraries
- DOM Libraries
- jQuery
- jQuery UI
- jQuery Mobile
- MVC Frameworks
- Backbone.js
- AngularJS
- EmberJS
- KnockoutJS
- Node.js
- MEAN Stack
- NPM
- Miscellaneous Libraries
- Modernizr
- Underscore.js
- Handlebars
- RequireJS
- TypeScript
- Debugging and Best Practices
- Debugging JavaScript
- Writing to the JavaScript console
- Setting breakpoints
- JavaScript strict mode
- ustom error handling
- JavaScript Best Practices
- Avoiding conflicts with JavaScript namespaces
- IIFE
- Tools
- Minification Tools
- JSLint
- JSPerf
- Tips and tricks
- Use shortcut notation
- Optimize loops
- Put scripts at the bottom of the page
- Find the most efficient way
- Unit Testing
- Jasmine
This course does not align to a specific exam or certification.
- For Private Groups as small as 2 people.
- Live, Instructor-led Online or Onsite Class for your group.
- Customizable to your needs.